Preparing for a software development interview involves several steps to ensure you are well-prepared and confident. Here’s a comprehensive guide to help you get ready:
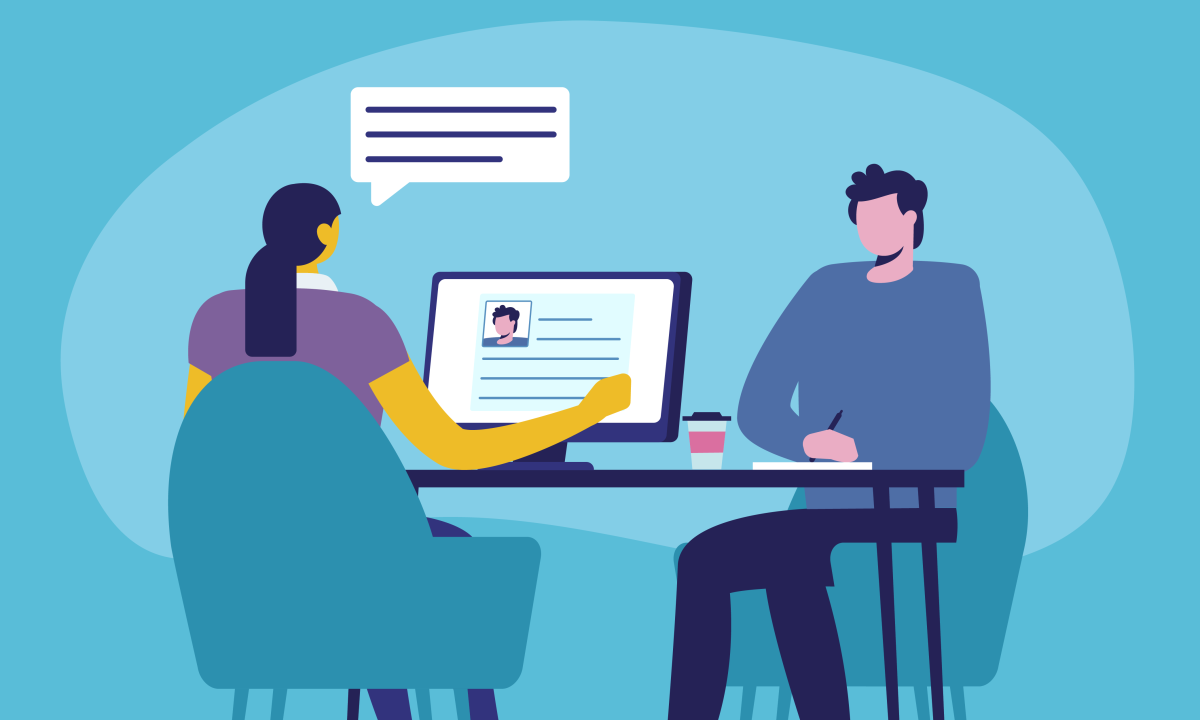
Understand the Interview Process
- Types of Interviews: Technical screen, coding test, behavioral interview, system design interview, and culture fit interview.
- Format: In-person, phone, video call, or online coding platforms.
Research the Company
- Company Background: Understand the company’s mission, values, products, and services.
- Tech Stack: Know the technologies and tools the company uses.
- Culture: Get insights into the company culture through Glassdoor reviews and LinkedIn.
Revise Core Concepts
- Data Structures: Arrays, linked lists, stacks, queues, trees, graphs, hash tables.
- Algorithms: Sorting, searching, dynamic programming, recursion, greedy algorithms.
- System Design: Design principles, scalability, load balancing, caching, database design.
- Programming Languages: Be proficient in at least one language relevant to the job (e.g., Java, Python, JavaScript).
Practice Coding
- Online Platforms: LeetCode, HackerRank, CodeSignal, Codewars.
- Types of Problems: Practice problems related to arrays, strings, trees, graphs, dynamic programming, and bit manipulation.
- Mock Interviews: Use platforms like Pramp, Interviewing.io, or pair up with a friend for mock interviews.
Behavioral Questions
- Common Questions: "Tell me about yourself," "Describe a challenging project," "How do you handle conflicts?"
- STAR Method: Structure answers using Situation, Task, Action, Result.
- Company Fit: Demonstrate how your values and experience align with the company’s culture and goals.
System Design
- Concepts: High-level design, scalability, load balancing, database partitioning, caching, CAP theorem.
- Practice: Design systems like URL shorteners, social networks, messaging systems.
- Resources: Books like "Designing Data-Intensive Applications" by Martin Kleppmann, "System Design Interview" by Alex Xu.
Technical Questions
- Coding: Write clean, efficient code with proper comments.
- Problem Solving: Explain your thought process clearly.
- Optimization: Discuss possible optimizations and trade-offs.
Revise Fundamentals
- OOP Principles: Encapsulation, inheritance, polymorphism, abstraction.
- Databases: SQL and NoSQL databases, query optimization.
- Networking: Basic networking concepts, RESTful APIs, HTTP/HTTPS.
Prepare Your Portfolio
- Resume: Highlight relevant experience, projects, and skills.
- GitHub: Showcase your projects and contributions.
- Portfolio Website: Create a website to display your projects, blog posts, and resume.
Ask Questions
- Role and Team: Understand the expectations, team structure, and growth opportunities.
- Work Environment: Ask about the company’s work culture, remote work policies, and work-life balance.
- Tech Stack: Inquire about the tools and technologies you’ll be working with.
Example Practice Questions
1. Coding:
- Reverse a linked list.
- Find the longest palindrome substring.
- Implement a LRU cache.
- Merge two sorted arrays.
2. System Design:
- Design a URL shortening service.
- Design a scalable chat application.
- Design an e-commerce platform.
- Design a file storage system like Dropbox.
3. Behavioral:
- Describe a time you had to learn a new technology quickly.
- Give an example of a challenging bug you fixed.
- How do you prioritize tasks when working on multiple projects?